Modding workflow
Creating new mods
Create a folder named “mods” in the game’s root folder. The game will load local mods from subdirectories in this folder.
E.g. to start work on a new mod, create the folder mods/MyNewMod, and start adding mod files to it. See further documentation on what files and data the game looks for.
Most data is reloaded when a scenario is started from the main menu, but adding new scenarios, paperdolls or resources requires a restart of the game.
Uploading to Steam
Once the mod is ready to be shared, copy its folder to another folder called steam_uploads (also located in the game’s root folder).
E.g. from mods/MyNewMod to steam_uploads/MyNewMod
This folder serves as a repository for uploading data to Steam and is not loaded by the game directly.
Optionally add PNG file called steamicon.png, which will be used as the icon for the mod on Steam Workshop.
Open the game and go to the Mods menu from the main menu. You will see a list of all the mods in steam_uploads. The first time you upload a mod, the mod will need to be initialized. Press the Init button, and a new file called modinfo.dat will be created in the mod’s folder. Do not delete or edit this file, as it contains important data connecting this mod to Steam. It cannot be recreated.
Once the mod is initialized and ready, the Upload button will be available, as well as title and description info. You can edit these fields and they will be updated on Steam Workshop when you upload. You should also add a message to describe what’s changed in this upload.
When you press the Upload button, the game will check that all files compile correctly and then start uploading to Steam. Hopefully everything goes well, and then a success message will be displayed. If anything went wrong, an error message will be displayed instead.
Then what?
Continue working on your mod in mods/ folder and when new changes are ready to be uploaded, copy the contents to its counterpart in steam_uploads, but make sure not to overwrite modinfo.dat.
To make a mod visible to others, you need to go into Steam Workshop and change its visibility. You can also edit descriptions and titles there, though note that those changes will not be downloaded back to steam_uploads and may be overwritten the next time you upload.
Scripts – Basic syntax
Line comments
// this is a line comment, anything after the two slashes will be ignored --- this is also a line comment
Instructions (<instr>):
<instruction> <argument0> <argument1> ... <argumentN>
e.g.
setlocal alpha 555
exception:
if arguments are expressions (see below), they need to be separated by a comma
currently this only applies to one instruction: add_stat, which takes a string
expression and an int expression as arguments
e.g.
add_stat "vio", 5+rand(3)
newlines separate instructions
exception: if, while, else, and blocks {, }, do not require newlines for the instruction that follow
see section: Basic Statements
Values:
Values of script variables can currently have 3 types
(Only the decimal form is currently supported)
Comparisons are only valid between types of the same kind
e.g.
5 = "5"
is always false
Operations always result in the same type as the first operand, and the second will be converted to this type of possible
e.g.
Expressions can be used as arguments to instructions to perform calculations
Any number of operators and comparisons can be combined anywhere that an expression is expected
They can use any of the following as operands
variables: an unquoted string, e.g., alpha. Will load the value of a previously defined script variable or a built-in value (see later) The order of precedence for loading values is 1. local variables (defined with setlocal) 2. script variables (defined with setvar) 3. built-in variables 4. globals (defined with global) literals: integers, e.g. 5 strings, e.g. "abc" lists, e.g. {5,5,5,5}
Sub-expressions can be grouped with parentheses
e.g.
(alpha + beta) * gamma alpha + (beta * gamma)
Available operators
(a and b are operands, they can be either named variables or literals)
Prefix operators:
+a (does nothing) -a negation negates a value, e.g. turns 5 into -5. Only valid for ints !a boolean inversion turns a boolean true (1) into false (0) and vice versa Only valid for ints
Arithmetic operators:
a * b multiplication multiplies a with b, e.g. 5*2 => 10 Only valid for ints a / b division divides a with b, e.g. 10 / 2 => 5 Only valid for ints a + b addition Ints: adds a and b, e.g: 5+2 => 7 Strings: appends b to the end of a, e.g: "abc" + "d" => "abcd" Lists: appends b as the last element of the list, e.g: {1,2,3} + "hello" => {1,2,3,"hello"} a - b subtraction subtracts a and b, e.g. 10 - 2 => 7 Only valid for ints
Ordering operators
(operands must be of same type)
(not defined for lists)
a < b "less than" comparison Ints: 1 if a is less than b, otherwise 0, e.g. 10 < 5 => 0 Strings: calculates lexical string ordering, e.g: "a" < "b" => 1 a <= b "less than or equal to" comparison Ints: 1 if a is less than or equal b, otherwise 0, e.g. 10 <= 10 => 1 Strings: calculates lexical string ordering, e.g: "b" <= "a" => 0 a > b "greater than" comparison Ints: 1 if a is greather than b, otherwise 0, e.g: 10 > 5 => 1 Strings: calculates lexical string ordering, e.g: "a" > "b" => 0 a >= b "greater than or equal to" comparison Ints: 1 if a is greater than or equal to be, otherwise 0, e.g: 10 >= 10 => 1 Strings: calculates lexial string ordering: e.g: "b" >= "a" => 1
Comparison operators
(always false if operands are not of same type)
a = b equality comparison 1 if a and b are exactly equal, otherwise 0 Lists: checks that each element of the lists are equal a != n inequality comparison 1 if and b are not exactly equal, otherwise 0 Lists: checks if any of the elements of the lists are not equal
Logical operators
(interpret their operands as boolean values (true/1 or false/0))
(non-zero ints are considered true, zero is false)
(non-empty strings are considered false, otherwise all strings are true)
(non-empty lists are considiered false, other all lists are true)
a && b logical AND 1 if both a and b are true, otherwise 0 a || b logical OR 1 if either a or b are true, 0 if both are zero
Index operator
a[b] gets the value at index b of a Ints: not valid Strings: returns the character value at the specified index as an int Lists: returns the value at the specified index
Scripts – Additional expressions
Function calls:
function_name(<arglist>)
calls the built-in function function_name with the specified arguments (see below for <arglist> definition)
trying to call an unknown function will result in a compile error
e.g: rand(5), returns a random value between 0 and 4
see section “Built-in functions” for full list of available functions
Select:
[<expr>: <arglist>]
selects any of the specified arguments based on the value of the <expr> expression
e.g:
[rand(3): "a", "b", "c"]
will result in either “a”, “b” or “c”, based on the value of rand(3)
conditional operator (<bool expr> ? (a) : (b)) can be implemented as [<bool expr>: a, b]
if <expr> results in a value that is outside the range of the arglist (less than 0 or larger than the number of items)
a warning will be issued and the first item chosen
shorthands:
a|b|c
shorthand for [diff: a,b,c]
a\b\c
shorthand for [q_diff, a,b,c]
Argument list expression (<arglist>)
Argument lists are used in function calls, list literals, and certain other contexts
They are a list of expressions separated by commas; <arg0 expr>, <arg1 expr>, <arg3 expr>
e.g.
func(1,2,3)
1,2,3 is the <arglist> here
they are usually enclosed by parentheses, but in list by brackets [],
and in select expressions, the square bracket ends the list
List expansion operator (…<expr>)
This operator expands a list value into additional individual arguments in an <arglist>
it is only valid in places that accepts an <arglist>
e.g.
setlocal arr {1,2,3} func(...arr)
will be interpreted as func(1,2,3)
as arr is a list variable
using the operator on a non-indexable value is an error
using it on a string will result in a list of character values
e.g.
..."abc"
may result in {97,98,99}
Scripts – Statements
A statement is one of the following
- <instr> – an instruction
- if – an if statement
- while – a loop statement
- end – a return statement
- @/label – a label statement
- jump – a jump statement
- {<stmt>…} – a block statement; multiple statements enclosed in brackets
Basic Statements
If
if <bool expr> <stmt>
if statement
executes the statement <stmt> if <bool expr> is true/1, otherwise doesn’t and skips to the statement after the if block
e.g.
if alpha = 5 dbg_log("hello")
only executes dbg_log if alpha is 5
for multiline ifs, use block statements
if alpha = 5 { dbg_log("hello") dbg_log("world") }
can be nested to to any depth
e.g.
if (alpha = 5) if (beta = 4) if (gamma = 3) dbg_log("yup")
only executes dbg_log if alpha is 5, and beta is 4, and gamma is 3
(this could of course also be written as if (alpha = 5 && beta = 4 && gamma = 3) )
If-else
if <bool expr> <"then" stmt> else <"else" stmt>
if-else statement
executes <then stmt> if <bool expr> is true/1, otherwise executes <else stmt>
if <then stmt> is executed, <else stmt> is skipped
e.g.
if alpha = 5 dbg_log("hello from 5") else dbg_log("hello from something else")
as with any statement, use block statements for multiline ifs or else blocks
Looping
while <bool expr> <stmt>
loop statement
executes <stmt> repeatedly as long as <bool expr> is true/1
use block statements for multiple instruction etc. etc.
End
end [<expr>]
return statement
ends execution of the current script
if the current script has been started with call, returns control to the calling script
can push value of the optional <expr> as a return value to the caller
Label
@<labelname>
or
label <labelname>
label statement
creates a new label named <labelname> that be jumped to, or be the target of other jumping instructions
only valid in the current script context
label names must be unique
unused labels will result in a compile error
Jump
jump <labelname>
jump statement
moves execution from the current position to the instruction following the label <labelname>
e.g.
@branch1 ... jump branch1
goes back and runs the code from branch1 again (beware of infinite loops when jumping upwards)
<labelname> can also be a select statement, by the <arglist> must consist of valid labels
caution: excessive jumping makes code hard to follow and reason about,
prefer using other methods of structuring code
Set variable
setvar <varname> <expr>
set script variable statement
sets a variable named <varname> to the value of <expr>
this value is available throughout the whole script
e.g.
setvar alpha rand(4) * (beta + gamma)
shorthands:
addvar <varname> <expr> => setvar <varname> (<varname> + (<expr>))
adds <expr> to the current value of <varname>
subvar <varname> <expr> => setvar <varname> (<varname> - (<expr>))
subtracts <expr> from the current value <varname>
Set local variable
setlocal <varname> <expr>
set local variable statement
sets a local variable named <varname> to the value of <expr>
this value is only available in current script context (event),
not in other events, not even if they have been goto’d or call’d
e.g.
setlocal alpha "hello " + "world"
shorthands
addlocal <varname> <expr>
same as addvar, but for local variables
sublocal
same as subvar, but for local variables
Debug log
dbg_log(<arglist>)
debug log statement
prints the arguments in <arglist> to the debug log
Scripts – Built-in functions
These can be used in expressions.
special values:
“gear” – checks if the player has an item of type weapon, armor, or relic
“weapon”/”magic”/”armor”/”relic”/”mist” – checks if the player has an item of this item type
can be used with e.g. pl_port to set the portrait of the player of a certain rank
pl_port ranked_player(2)
sets the portrait to player of rank 2
Scripts – Built-in variables
These can be used in expressions.
tou
mag
sne
soc
coo
Scripts – Script instructions
These instructions handle how scripts are parsed or define new events. strict
legacy
changes the parsing mode of the parser
these are only valid outside of events and will be interpreted as the end of the event if present inside an event
global <varname> <expr>
defines a new global variable named <varname> with the value <expr>
only valid outside of events and will be interpreted as the end of an event
<expr> is executed during compile time and in the order defined; beware of using variables other than earlier globals in <expr>
id: <event id> [override]
defines a new event named <event id>
the id must be unique, if the event already exists in the base game you will get an error
if you really want to override an existing script, mark the event as override.
e. g.
id: event_that_exists override
if no such event actually exists to override, you will instead get an error for that.
Scripts – Event instructions
The following instructions are only valid inside an event goto <event id> [(<arglist>)]
starts the event <event id> and changes context there
if the optional <arglist> is specified, these values will be available in the goto’d event as local variables
argument variables are named
argv: a list of all the arguments
arg_0 … arg_N: named variables of the values in the order defined in <arglist>
argc: number of arguments passed to event
e,g,
goto test_event (1, 2, 3)
test_event will have local variables
argv = {1, 2, 3}
argc = 3
arg_0 = 1, arg_1 = 2, arg_2 = 3
<event id> can also be a select statement, but the results must be valid event ids and cannot be full expressions
call <event id> [(<arglist>)] [<retval>]
same goto, but when <event id> ends, control returns to the current event and continues with the next instruction
after call
if <retval> is specified, a local variable named <retval> is stored with the value of the return value
e.g.
call test_event rval dbg_log(rval) id: test_event end 5
dbg_log will display 5, as that was the value returned from test_event and stored in rval
rng <int = N>
<stmt 0>
…
<stmt N-1>
defines a random switch statement
selects a random value between 0 and N and executes one of the following N instruction based on that value
e.g.
rng 3 dbg_log("selected 0") dbg_log("selected 1") dbg_log("selected 2")
will execute one of the dbg_log statements
the others are skipped
as with other <stmt>, block statements are considered a single statement
rng <instr type>
NOTE: Preferably don’t use this one
similar to rng, but reads all the immediately following instructions of type <instr type>
and uses them in the list of random instructions to execute
e.g.
rng jump jump label1 jump label2 jump label3 @label1
will use one of the random jump instructions between rng and label1
ch_if <bool expr>
ch_0 <line>
ch_1 <line>
ch_2 <line>
ch_str <string expr>
NOTE: Wonky legacy syntax here
choice statement, displays a choice box, with a number of options
any of these initiates parsing of a choice statement
ch_0, ch_1, ch_2
Pushes the line as a new choice option
ch_str
Takes a string expression and pushes the value as a new choice option
ch_if
Make the next ch_X statement conditional on the value of the <bool expr>
If it is false/0, that option can’t be selected
If the value of ch_X is -, that option is ignored
NOTE: This is not necessary and only available for legacy reasons
If you only want two options, then you only need to write two ch_X instructions
text in choice boxes is filtered, see section String Filtering
choice
acts as break between ch_X and the results of the choices
reads as many <stmt> after choice as the number of ch_X lines (ie. ch_if does not count to choice count)
that is, with two choices, it will read two <stmt>s and execute the one chosen in the box
e.g.
ch_0 Choice 1 ch_str "Choice 2" choice dbg_log("chose 1") dbg_log("chose 2")
displays a choice box with two options: “Choice 1” and “Choice 2”.
Depending on which is chosen, either of the two dbg_logs are executed
Statements not chosen are skipped
As with other <stmt> block statements can be used for multiline instruction results etc. etc.
endturn
removes all remaining moves of the current player and skips the “resolve tile” step of the turn
meaning no other events would be drawn
exhaust
removes all remaining moves of the current player
add_ee <event id> [<labelname>]
adds the event <event id> on the map the next time the game returns to the map
if the enchantment event can’t be placed on the current tile the script jumps
to the optional label <labelname>, if specified
fg_ev <event id>
do this before “fight” to add an override after-winning-event to the fight.
this will ignore any after-winning-event set in the monster data.
this override will remain with the monster on the map.
(if you want an event when you lose a fight, you add it in the monsters data:
– lose_ev: <event id> )
start_fight <string expr>
start a fight with a monster with id of the value of <string expr>
e.g.
start_fight "monster_id"
starts a fight with the monster monster_id
shorthands
fight <monster id> => start_fight "<monster id>"
start_fight_msg <string expr>
works like start_fight except it shows a random encounter message in the beginning of combat
block_check
shortcut to check levels based on area [#blockerlvls]
and does a goto: to [#monster_lvlblockers] if level is not high enough
Scripts – Event instructions (quests)
sets the name of the quest to <line>
any event that has a q_nm command in its body can’t be drawn if an
active quest has the name <line> or if a quest in its line has the name <line> q_ev <event id>
sets the quest event to <event id>
q_rew <reward id>
sets the quest reward to <reward id>
also accepts select literals
q_mr <int expr>
value of <int expr> = minimum range (number of tiles) away the quest objective has to be
sets the minimum range for the quest objective
q_diff <int expr>
if you set <int expr> to anything over 0, the next add_quest or add_quest all will place the quest somewhere in the region of that difficulty
but if you set this to 0, the quest will be placed in your current region or below
q_area <area id>
<area id> = darklair / castle
places the next add_quest or add_quest_all in this specific area.
other areas than darklair and castle will show an error
<area id> = NULL disables this feature and places quests normally, only needed if you place more than one quest in one event
q_req <line>
explanation of requirement to do the quest event
displays <line> as text in the quest menu
add_quest [<mode>]
adds a new quest and spawns the event in an appropriate place.
relies on q_nm and q_ev and q_rew being set beforehand.
if this event was the “next event” of a quest, that quest is replaced with this new one.
optional <mode> =
- all, adds quest for all players
- main, adds as main quest
shorthands
add_quest_all => add_quest all add_quest_main => add_quest main
Scripts – Event instructions (misc)
wait for <expr> / 50 seconds before running the next instructions
e.g.
wait 25
waits for 0.5 seconds
wait_for_input [<varname>]
waits until the player presses a button
if <varname> is specified, the value of the pressed button is stored in the local variable <varname>
death_check
checks if the player is dead, and if so ends the script
Scripts – Event instructions (text)
clears the message box nm_clear
clears the name box
name <string expr>
displays the value of <string expr> in the name box
shorthands
nm <line> => name "<line>" nm_p => name pl_name display the current player name nm_n <string> => name get_name("<string>") displays a name string <string> nm_m <monster id> => name monster_name("<monster id>") displays name of monster <monster id>
message <arglist>
displays the values of <arglist> in the message box
text in message box is filtered, see section String Filtering
box <line>
displays the line <line> as text in the message box
text in message box is filtered, see section String Filtering
nag <line>
works like the box command if the user has the Hint Messages setting on
otherwise this command is skipped
add <line>
adds the line <line> as text to the message box
text in message box is filtered, see section String Filtering
Scripts – Event instructions (misc. scene, graphics)
snok events MUST have this
reset the Snok counter which allows players to get a Snok encounter
must be called in every Snok event when the player gets the reward
if the group has Snok event group with 1 or more in event_groups.txt it’s allowed to draw it. Castle And Dark Castle have no Snok available, so it cannot be drawn there. next_act
makes the game move forward one act after the event.
run_scene <string expr>
runs an EventScene prefab from Resources/EventScenes/ named as value of <string expr>
shorthands
scene <scene name> => run_scene "<scene name>"
set_bkg <string expr>
sets the background image to the value of <string expr> (searches first in Assets/Resources/Backgrounds)
shorthands
bkg <image name> => set_bkg "<image name>"
bkg_restore
restores the background to the tile-appropriate one
set_port <string expr>
sets the portrait to the value of <string expr> (searches first in Assets/Resources/Portraits)
to clear, use img name “None”, which is a small 100% transparent texture
shorthands
port <image name> => set_port "<image name>" port_m <monster id> => set_port monster_gfx("<monster id>")
port_pl <int expr>
displays the portrait of player of index <int expr>
shorthands
port_rank_pl <expr> => port_pl ranked_player(<expr>)
displays the portrait of the player of a certain rank
Scripts – Event instructions (audio, effects, music)
show a portrait fx (sweat drop, anger thingy, shake etc). will be automatically cleared when changing portrait
these will stack so you can mix severalshorthands
fxp <fx> => play_fx "<fx>"
fxp_clear
remove all current portrait fx
play_music <string expr>
plays the song <string expr>
shorthands
music <name> => play_music "<name>"
set_next_music <string expr>
set the song <string expr> to be played next
shorthands
music_next <name> => set_next_music "<name>"
set_map_music <string expr>
sets the song <string expr> to be played on the map
shorthands
map_music <name> => set_map_music "<name>"
set_next_map_music <string expr>
set the song <string expr> to be played next on the map
shorthands
map_music_next <name> => set_next_map_music "<name>"
music_interrupt
instantly pauses the overworld music and instantly stops the “event music”.
music_fadeout
stops the event music with a fadeout
music_pause
pauses the event music
music_resume
resumes the paused event music
play_snd <string expr>
plays the sound effect <string expr>
this sound interrupts the previous event sound
shorthands
sfx <name> => play_snd "<name>"
play_snd_ducked <string expr>
(UNDER CONSTRUCTION)
plays a sound just like the play_snd command but
the music ducks this quickly and intensely
shorthands
dsfx <name> => play_snd_ducked "<name>"
Scripts – Event instructions (stats, items, jobs, rewards, etc.)
updates the stat <string expr> with the value of <int expr> (note comma separating the two expressions)
will display a message box notifying the player of the change
e.g.
add_stat "vio", 5+rand(3)
adds 5-7 to the stat VIO
shorthands
+vio <expr> => add_stat "vio", <expr> +tou <expr> => add_stat "tou", <expr> +mag <expr> => add_stat "mag", <expr> +sne <expr> => add_stat "sne", <expr> +soc <expr> => add_stat "soc", <expr> +coo <expr> => add_stat "coo", <expr> +rst <expr> => add_stat rand_entry(stats), <expr> updates a random stat
add_item <string expr>
gives the player a specific item
shorthands
+item <name> => add_item "<name>"
rem_item <string expr>
removes the item <string expr> if it exists in the players inventory
shorthands
-item: <name> => rem_item "<name>" NOTE: colon after -item differentiates it from -item below
rem_item_type <string expr>
removes an item of a specific type from the player’s invetory, if it exists
shorthands
-item => rem_item_type "all" NOTE: lack of colon differentiates it from -item: -weapon => rem_item_type "weapon" -armor => rem_item_type "armor" -relic => rem_item_type "relic" -magic => rem_item_type "magic" -gear => rem_item_type "gear"
add_exp <int expr>
updates the player’s EXP value with the value of <int expr> and displays a message notifying the player of the change
shorthands
+xp <expr> => add_exp <expr>
add_hp <int expr>
updates the player’s HP with the value of <int expr> and displays a message about this.
if HP drops below 0, the event ends immediately
shortands
+hp <expr> => add_hp <expr>
add_status <string expr>
adds the status <string expr> to the player
shorthands
+s <name> => add_status "<name>"
rem_status <string expr>
removes the status <string expr> from the player if he has it
no textbox is shown if the player doesn’t have the status effect
shorthands
-s <name> => rem_status "<name>"
status_clear
removes all the status effects from the players
automatically shows a textbox for every status removed
set_job <string expr>
changes the players job to <string expr> bypassing all normal requirements
shorthands
+job <name> => set_job "<name>"
give_reward <string expr>
gives the player a random item from the category in <string expr>
shorthands
+reward <name> => give_reward "<name>" +reward: #jobarea gives equipment tagged for the specific job the player currently is. If a new job is added, equipment must be tagged, or this function will crash the event.
Scripts – Event attributes
These look like instructions but are interpreted during compilation and added to an event as attributes freq <rarity>
<rarity> is a number typically from 2-8, or it can be rare (1), common (5), or uncommon (3)
sets how frequently an event appears
group <groupname>
sets which group an event belongs to
all events except those special or starter attributes must have a group
valid groups are:
- good (something good happens)
- bad (something bad happens)
- event (random chance events)
- snok (only appears for the player in last place)
- quest (events that start quests)
loc <location>
sets which location the event should appear in
an event can have multiple loc attributes and then it will appear in all those locations
valid loc are:
- road (generally very low risk and rewards, no quests)
- town (generally very low risk and rewards, no quests)
- flowers (generally find jobs)
- forest (generally find cash)
- ruins (generally find equipment)
- runestones (generally find spells)
- mountain (generally high risk and high rewards)
diff <range>
sets difficulty of the event
<range> can a single integer, or two integers separated by -, e.g. 0 – 3 (spaces are not necessary; negative integers are not accepted)
the first region is 0, the final region is 3
special
marks this event as special, it will not appear as a random event
pvp
marks this event as a pvp event, it will not appear as a random event if the player has disabled pvp events
enchantment <line>
sets the event as special and marks it be used for enchant events
<line> will be interpreted as a string and used as the name of the enchant event
enchantment_diff <int>
sets the tier of the area this enchantment would spawn in
0 is the default and means the current space when spawned in an event or a random space
if it is randomly placed by proceeding to a new act
enchantment_unremovable
makes this enchant event unremovable by other enchants (such as golden road)
put this after the enchantment: <string> portion in the event that is created to make it unremovable
starter
a possible starter quest that happens before the game
automatically sets event as “special” as well
lastplace_only
event can only randomly spawn for someone in last place
notfirst_only
event can only randomly spawn for someone in not in first place
first_only
event can only randomly spawn for someone in first place
notlast_only
event can only randomly spawn for someone not in last place
not_in_quests <event id list>
prevents a random event from being chosen if a quest with a q_ev found in <event id list> is currently active
e.g.
not_in_quests {event_1, event_2}
event ids are checked to make sure events with those ids actually exist
Note that when regular random quests are created in the games random events that event is already getting prevented from being picked again for as long as the quest remains on the quest log
Scripts – String filtering
These are enclosed in <variable> brackets
Any available script variable can be used and displayed in this wayThere are also some special values:
E.g.
box We are currently in <location>...
May display “We are currently in Freaky Forest…” in the message box.
Scripts – About events and event groups
A player who has been in last place for 3 consecutive turns (or 4 turns when there are only two players), and is a minimum of 2 levels behind the leading player, automatically gets a Snok event if one is available at that location.
All events have a cooldown of 8 turns before any player can draw them again. Quests have a cooldown of 25 turns.
The castle and darklair locations have a special case; they only contain the events defaultcastle and defaultdarklair respectively.
When a player gets a random event, the event group is chosen first, and then a specific event is chosen within that group.
The Clairvoyant skill has a chance of rerolling the event group once if the Bad group was drawn. The Lucky status forces the Good or Quest group to be drawn, and the Unlucky status forces the Bad group to be drawn.
This is the frequency of each group of random events when exploring/loitering on each location type per region:
Data formats – Common
Data specified in square […] brackets are optional, and it is not an error to leave them out. Some default value will be used instead.
Values specified as <…> means those values should be defined by you. The brackets should not be included in the actual data file.
E.g., if a specification says <resource id>, you could write my_very_cool_resource_id in the data file.
Data formats – Resources
(define all resources in this file)
File comments allowedResources are loaded when needed, that is, if a resource is defined but never used, it will not get loaded.
All paths should be considered local to the root folder of the mod.
Resource ids can in some cases override “hardcoded” resources, ie. those that come built-in with the game. This can be done for e.g. any texture referenced by scripts, or enemy sprites. To always use a certain built-in resource, reference it as res:/<resource id>
Format:
[Textures] tex <texture id> { path: <local path of texture file> }
Note: Valid file formats are png and jpg
[Sprites] sprite <sprite id> { path: <local path of texture file for sprite> [pixels_per_unit: <scale value; default: 128>] [pivot_x: <horizontal center offset in pixels>] [pivot_y: <vertical center offset in pixels>] }
Notes: Same file formats as for textures
[Music] music <music id> { path: <local path of texture file for sprite> [loop_left: <time in ms; default: 0>] [loop_right: <time in ms; default: 0>] [next: <music id>] }
Notes:
The song will loop if loop_left is a smaller value than loop_right.
The ‘next:’ variable only matters if this song is used as the overworld song.
When a new act begins the game checks the ‘next:’ variable for what song to switch to.
Example:
tex TestMod_apamouth { path: textures/apa_mouth.png } sprite TestMod_Sprite1 { path: textures/sprite1.png pixels_per_unit: 75 pivot_y: -70 }
Data formats – Paperdoll
(define all new doll parts in this file)
File comments allowedAdds new selectable mouths, hairstyles, etc., for the player sprites
Format:
<doll part type> <part id> { gfx: <texture id> [tag: <tag id>] }
Available <doll part type> that can be selected:
- hair_back – piece of hair rendered behind head
- head – the sprite’s basic head shape
- mouth – the mouth
- eyes – eyes
- hair_front – piece of hair rendered in front of the head
Notes:
Tags are an optional feature that determine which parts that are incompatible with one another.
Currently only used for hairs. If a hair_front part has a tag, a hair_back needs to have the same tag in order to be used with that hair_front. Otherwise it will be skipped when trying to select it in the menu.
Example:
head apafrog { gfx: TestMod_apahead } eyes apaeyes { gfx: TestMod_apaeyes } mouth apamouth { gfx: TestMod_apamouth }
Data formats – Scenario
(define all new scenarios in this file)
File comments allowedDefines new scenarios that can be selected in the game menu.
Format:
{ name: "<name of the scenario to be displayed in the menu>" [desc: "<longer description of the scenario>"] start_event: <script event id that should start the scenario> [boss_exp_multiplier: <amount of EXP awarded per 100 HP damage dealt to the final boss, default: 333>] [final_reward_exp: <EXP reward for random bonuses at end of game, default: 750>] [start_gold: <how much cash to start the game with>] [turn_counts: <list of four integers that defines how many turns there should be for 1-player games, 2-player games etc, default: 50 48 42 38>] timeup_event: <script event id that should play when the number of turns are up> [music: <id of basic scenario music>] [enchant_events: <list of enchantment script event ids>] [act_settings: { {<list of settings for act 1>} {<list of settings for act 2>} {<list of settings for act 3>} {<list of settings for act 4>} }] [map_settings: { area_tiles: <list of 4 integers that define the number of tiles per region, must add up to 64, default: 12 15 17 20>] max_deadend: <integer for max length of dead ends, default: 2> max_path: <integer for max distance between two neighbouring tiles, default: 7> num_locations: <list of 4 integers for number of locations per region, must be at least 2 1 1 2, default: 4 5 6 7> num_locations_add: <list of 4 integers for the maximum number of locations to add randomly to each region, default: 1 1 1 1> }] [waste_events: <0 to disable, 1 to enable, default:1>] [snok_events: <0 to disable, 1 to enable, default:1>] [pvp_events: <0 to disable, 1 to enable, default:1>] [final_rewards: <0 to disable, 1 to enable, default:1>] [gold_rate: <integer as percentage, default:100>] [exp_rate: <integer as percentage, default:100>] }
Possible act settings:
- spawn_bags – spawn chests with cash and EXP during the act
- monster_surge – enable monster surge event
- extra_move – enable +1 extra moves per turn
- teleporter – enable a randomly placed teleporter
- music – enable music
- enchant – enable enchantment events
Example:
{ name: "TestMod Scenario" desc: "A scenario from the mod TestMod.\n\nYep." start_event: testmod_event_start start_gold: 500 turn_counts: 50 48 42 38 timeup_event: testmod_timeup enchant_events: expertised_enchantment speedy_enchantment act_settings: { {} {spawn_bags extra_move music} {spawn_bags teleporter music enchant} {spawn_bags monster_surge extra_move music} } }
Data formats – Constants
(define all constants in this file)
File comments allowedConstants are single expression shorthands that can be used as values in scripts. Expand constants in scripts with #
Format:
<constant id> <constant value>
Example:
my_cool_constant 4|5|6|7 // Defines a new constant "my_cool_constant" with the value 4|5|6|7
Using the constant in a script:
Data formats – Names
(define all names in this file)
File comments allowedDefines named strings that can be used in scripts, by e.g. the functions get_name() or event nm_n
Format:
name: <value of new name string>
Example:
id: apafrog
name: Apathetic Frog
Using a name in a script:
name get_name(“apafrog”) // Does the same thing
Data formats – Jobs
(define all classes in this file)
File comments allowedDefines new jobs that players can use.
Format:
id: <id of new class; marks the start of new class definition> name: <string line for the in-game name of the class displayed in menus etc.> [gfx: <resource id of the player sprite gfx for the job, e.g. "hat", default: job_<id> >] [reorder_gfx: <set to 1 to use special render ordering of the gfx>] [town: <list of ints for towns in which the class should be found>] [req_vio: <minimum VIO needed for the player to buy this class>] [req_tou: <minimum TOU needed>] [req_mag: <minimum MAG needed>] [req_sne: <minimum SNE needed>] [req_soc: <minimum SOC needed>] [req_coo: <minimum COO needed>] [cost: <how much money it costs to use the class>] [skill: <adds a skill the player can use when having the class; not a list, add multiple skills by having multiple skill: statements>]
Example:
id: delinquent name: Delinquent town: 1 // Available in level 1 town // No gfx: define, uses gfx job_delinquent reorder_gfx: 1 // Render gfx behind hair_front req_coo: 3 cost: 120 // The class gives both "knifecrimes" and "mug" skills skill: knifecrimes skill: mug
Data formats – Items
All .txt files in the items folder will be parsed as item definitions
File comments allowedDefines new items; weapons, armors, accessories etc., that can be held and used by players.
Format:
id: <id of new item; marks the start of a new item definition> name: <line string of display name of item> type: <type of item> [desc: <line string of description of item>] [gfx: <resource id of gfx the item puts on the player sprite; defaults depend on item type, <type>_<id> >] [spell: <id of spell the item uses>] [wanted_by: <list of class ids that the item is wanted by, which adds it to some rewards tables>] [+dmg: <extra damage the player does while equipping the item>] [+acc: <extra accuracy>] [+def: <extra defense>] [+mdef: <extra magic defense>] [+vio: <extra VIO>] [+tou: <extra TOU>] [+mag: <extra MAG>] [+sne: <extra SNE>] [+soc: <extra SOC>] [+coo: <extra COO>] [cost: <cost to buy item>] [sell: <selling price of item, default: cost / 2>] [skill: <add a skill that is gained while equipping item>] [subtype: <subtype of item>]
Item types:
- weapon – Weapon
- armor – Armor
- relic – Accessory
- magic – Spell
- misc – Misc
Weapon subtypes:
- stick
- knife
- snake
Example:
id: doubleedge name: Double edge desc: Twice the knife. type: weapon subtype: knife wanted_by: none cost: 1300 +dmg: 65 +acc: 10
Data formats – Monsters
(all .txt files in the monsters folder will be parsed as monster definitions)
File comments allowedDefines new monsters that can be encountered in the world or battled via scripts.
For “percentage” values, an optional % can be added after the value, which is ignored during parsing.
For “range” values, either a single integer, or two integers separated by a – can be used, e.g. a range of 2 – 5 defines a range between 2 and 5 and a range of 4 defines a range between 4 and 4.
Format:
id: <id of new monster; marks start of new monster definition> name: <line string of monster name, displayed in-game during battles etc.> gfx: <resource id of gfx sprite for the monster> [sneak: <percentage chance of successfully sneaking past monster>] [escape: <chance of successfully fleeing from an encounter>] hp: <how much HP the monster has> [hitability: <anti-dodging percentage, or rather how easy it is to hit the monster>] [def: <how much defense the monster has>] [mdef: <magical defense>] [fire: <elemental resistance to fire; -1 = weak, 0 = no change, 1 = strong>] [ice: <elemental resistant to ice>] [cute: <elemental resistant to cute>] [nasty: <elemental resistant to nasty>] [hitchance: <percentage of enemy attacks that hit player>] [critchance: <percentage of hits that will be critical hits>] [boss <flag monster as a boss, which gives it a special background and music>] [skip_ending <flag to skip the normal battle ending that plays a fanfare and gives you rewards>] [dmg: <range of damage for monster attacks>] [gold: <cash reward for defeating monster>] [exp: <EXP reward for defeating monster>] [win_ev: <script event id for event to run when winning against monster>] [lose_ev: <script event id for event to run when losing fight>] [esc_ev: <script event id for event to run when fleeing fight>] [skill: <add a skill the monster has>] [encounter: <list of area levels the monster can be encountered in>] [spell: <add a new spell the monster can use, max 4> >chance: <percentage chance to use the spell> >dmg: <range of much damage the spell does> >recharge: <how many battle turns before the spell can be used again> >status: <percentage chance of inflicting status> <sets the range of the buffing amount for the status effect, only applies to Pumped, Bricked and Insulated> ]
Example:
id: kaisernova //unique id name: Kaiser Nova gfx: kaisernova - encounter: 4 //encounter in level 4 areas sneak: 5 % //chance to sneak past (these "%" do nothing, just for readability) escape: 10 % //chance to escape battle hp: 390 //max HP hitability: 45 % //anti-dodging, or rather how easy it is to hit the monster def: 40 //defense mdef: 20 //magic defense fire: 0 //elemental def. -1 weak, 0 normal, 1 strong (and status immune) ice: 0 //elemental def. -1 weak, 0 normal, 1 strong (and status immune) cute: 0 //elemental def. -1 weak, 0 normal, 1 strong (and status immune) nasty: -1 //elemental def. -1 weak, 0 normal, 1 strong (and status immune) hitchance: 110 % //chance to land attack critchance: 10 % //chance to do critical damage dmg: 45 - 60 //range of damage - spell: heartbreak //has this spell, max 4 spells >chance: 35 % //chance to cast the spell >dmg: 45 - 65 //override base dmg/heal with this >status: 60 % 0 - 0 // chance to status hit, effect of status >recharge: 100 //cannot use spell again for this many turns - spell: flirt //has this spell >chance: 35 % //chance to cast the spell, max 4 spells >dmg: 65 - 80 //override base dmg/heal with this >status: 80 % 0 - 0 // chance to status hit, effect of status >recharge: 100 //cannot use spell again for this many turns - exp: 1390 //exp reward gold: 1250 //cash reward
Data formats – Events
(all .txt files in the events folder will be parsed as script files defining events)These files define new events that can be run in the game as scripts.
See Scripting documentation for reference.
(all new rewards should be defined in this file)
File comments allowedDefines new reward categories that can be used in scripts as rewards.
A reward category is basically a list of one or more items; when given as reward, a random item from the list will be chosen.
Format:
<reward id, defines start of new category>: <item id> [<item id>] [<item id, etc.>]
Example:
// Defines the new reward category "t1_weapon" t1_weapon: bluntsock twig butterknife fakesnake newspaper foamstick knice biggnukkel toysword
Using a reward table in script:
give_reward “t1_weapon” // Same as above
Resource lists – Portraits
These are all the portraits in the base game. You can refer to them when scripting your events etc. Please see the scripting documentation for more information.
Click the pictures to zoom in.
Resource lists – Backgrounds
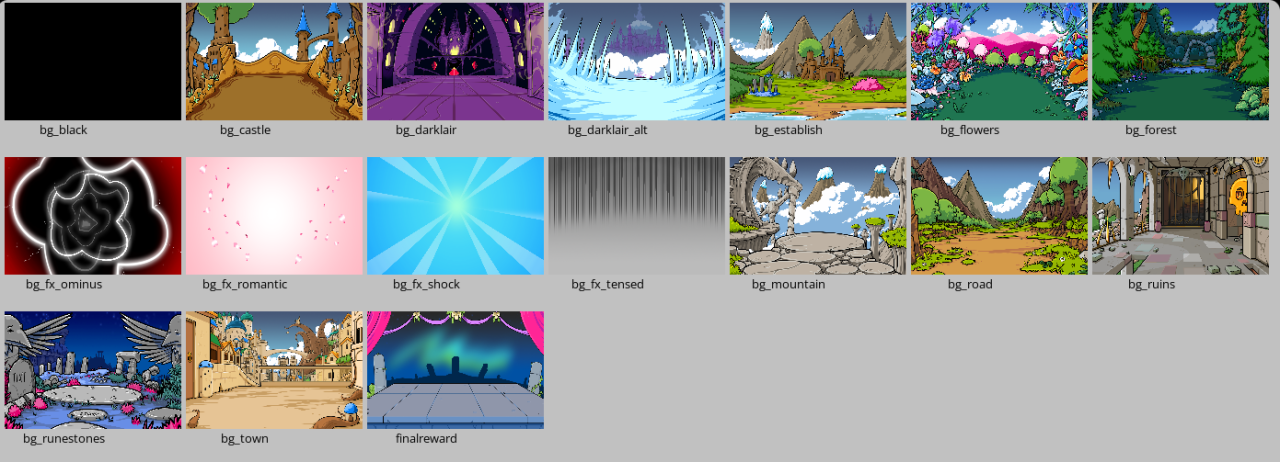
There are some special backgrounds in the game, but they have the wrong aspect ratio and thus will look weird when used in events. If you want to use them anyway, here they are:
Resource lists – Effects
All the effects in the base game.
Effects used with the fxp: command. They also play a sound effect.
Note: confetti and confetti2 are the same visually, but use different sound effects.
Note: evilexplosion and evilpuff are roughly the same visually, but use different sound effects.
Note: exclamation is to be used at the start of events that lead to quests.
Click the picture to zoom in.
Resource lists – MoveFX
These effects are used by combat skills, followers, spell casting, etc. and are only intended for such use, NOT event scripting.
AccessoryDebuff
BattleBadHit
BattleCrit
BattleHit
BattleMiss
BattleResistedCute
BattleResistedFire
BattleResistedIce
BattleResistedNasty
BattleShake
FollowerAnnebiguous
FollowerAvengingspirit
FollowerBombus
FollowerChampion
FollowerClog
FollowerFurnacecannon
FollowerPompom
FollowerSchoolclass
FollowerSleepwalker
FollowerSleuth
MapCollectTreasure
MapSpellBootGoop
MapSpellBootGoopInflict
MapSpellExperienceMagnet
MapSpellGeneric
MapSpellGenericNegative
MapSpellGoldenRoad
MapSpellGoldenRoadReceive
MapSpellGoldenRoadStepOn
MapSpellHealing
MapSpellHomingMagpie
MapSpellMonsterWhistle
MapSpellRinsingBath
MonsterSkillBossTransform
SkillAnalyze
SkillAnalyzeComplete
SkillBandAid
SkillCrush
SkillDoubleEdge
SkillDrainDamage
SkillDrainHeal
SkillGratingVoice
SkillMug
SkillPennyPinch
SkillRiskyMoves
SkillUnluckyStart
SpellAntiMagicSurge
SpellAntiMagicSurgeDamage
SpellAstralFlex
SpellAstralFlexInflict
SpellBattleTraining
SpellBattleTrainingDamage
SpellBrickPile
SpellBrickPileDamage
SpellColdFront
SpellDarknessFalls
SpellDistractions
SpellDistractionsInflict
SpellEncouragingWords
SpellEncouragingWordsDamage
SpellEvilPhenomena
SpellFlashFire
SpellFlirt
SpellFlirtInflict
SpellFullHeal
SpellGoldRush
SpellGoldRushDamage
SpellHardenSteel
SpellHardenSteelDamage
SpellHealing
SpellHeartBreak
SpellHeartBreakInflict
SpellHugToDeath
SpellHugToDeathInflict
SpellIceAge
SpellIceBlizzard
SpellImminentBeatdown
SpellImminentBeatdownInflict
SpellIncinerateStatus
SpellIncinerateStatusInflict
SpellJinx
SpellKnockout
SpellKnockoutInflict
SpellLightlyBroiled
SpellLuckyBreak
SpellMusclePump
SpellMusclePumpDamage
SpellPeekABoo
SpellPillowCannon
SpellPollution
SpellPowerWalk
SpellRinsingBath
SpellRinsingBathDamage
SpellRuseOutrage
SpellRudeOutrageInflict
SpellSlipperyCold
SpellSlipperyColdInflict
SpellSmokeBomb
SpellSnakeHeads
SpellSolarOven
SpellSparkles
SpellStayCool
SpellStayCoolInflict
SpellTacticalRainbow
SpellWeakenToCute
SpellWeakenToCuteInflict
SpellWeakenToFire
SpellWeakenToFireInflict
SpellWeakenToIce
SpellWeakenToIceInflict
SpellWeakenToNasty
SpellWeakenToNastyInflict
Resource lists – Names
All the name definitions in the base game.
Resource lists – Sounds
All the sounds in the base game. Used with the sfx: command.
BattleBadHit
BattleCrit
BattleEnemyDefeated
BattleHit
BattleMiss
BattleResistedCute
BattleResistedFire
BattleResistedIce
BattleResistedNasty
BattleTransition
EventApplause
EventBad
EventBadStatus
EventBigDoorSlam
EventBrickBreaking
EventCards
EventCheer
EventCheer2
EventChoiceMade
EventCrowdBoo
EventCrowdCheer
EventDoor
EventDrumrol
EventExplosionMagic
EventFall
EventFallTumble
EventFootsteps
EventFormlessExplosion
EventGainExp
EventGainGold
EventGainHP
EventGainItem
EventGainStat
EventGood
EventGoodStatus
EventHammer
EventHauntingEffect
EventIceExplosion
EventLaugh
EventLightsOut
EventLoseExp
EventLoseGold
EventLoseHP
EventLoseItem
EventLoseStat
EventMystery
EventNextAct
EventOhNo
EventOminous
EventOpenChest
EventPaper
EventPop
EventPotentialQuest
EventPressure
EventQuestComplete
EventScenarioWon
EventSmash
EventSmashEcho
EventSplashBig
EventSplashSmall
EventSpooky
EventStatusClear
EventStingerDramatic
EventStingerHappy
EventSurprise
EventSweat
EventSwosh
EventTakeDamage
EventTeleport
EventTimeUp
EventWhistleLong
EventWhistleShort
FollowerAnnebiguous
FollowerAvengingspirit
FollowerBombus
FollowerChampion
FollowerClog
FollowerFurnacecannon
FollowerPompom
FollowerSchoolclass
FollowerSleepwalker
MapChoicePopup
MapCollectTreasure
MapEnterTown
MapJoinBattle
MapMove
MapNewDay
MapOverview
MapPlaceBag
MapPlaceEnchantmentEvent
MapPlaceMonster
MapPlaceQuest
MapPlaceTeleporter
MapRespawn
MapSneakFailed
MapSneakSuccessful
MapSpellGeneric
MapStay
MapTileFlip
MapUndo
MapUseTeleporter
MenuBuy
MenuEquip
MenuError
MenuHospitalHeal
MenuNo
MenuOpen
MenuSell
MenuStartGame
MenuTab
MenuTick
MenuYes
MonsterSkillBosstransform
PlayerJobChange
PlayerLevelUp
SkillAnalyze
SkillAnalyzeComplete
SkillBandAid
SkillCrush
SkillDoubleEdge
SkillDrainDamage
SkillDrainHeal
SkillGratingVoice
SkillMug
SkillPennyPinch
SkillRiskyMoves
SpellAntiMagicSurge
SpellAstralFlex
SpellAstralFlexInflict
SpellBattleTraining
SpellBootGoop
SpellBootGoopInflict
SpellBrickPile
SpellColdFront
SpellDarknessFalls
SpellDistractions
SpellDistractionsInflict
SpellEncouragingWords
SpellEvilPhenomena
SpellExperienceMagnet
SpellFlashFire
SpellFlirt
SpellFlirtInflict
SpellFullHeal
SpellGenericDamage
SpellGoldenRoad
SpellGoldenRoadStepOn
SpellGoldRush
SpellHardenSteel
SpellHealing
SpellHeartbreak
SpellHeartbreakInflict
SpellHomingMagpie
SpellHugToDeath
SpellHudToDeathInflict
SpellIceAge
SpellIceBlizzard
SpellImminentBeatdown
SpellImminentBeatdownInflict
SpellIncinerateStatus
SpellIncinerateStatusInflict
SpellJinx
SpellJinxInflict
SpellKnockout
SpellKnockoutInflict
SpellLightlyBroiled
SpellLuckyBreak
SpellMonsterWhistle
SpellMusclePump
SpellPeekABoo
SpellPillowCannon
SpellPollution
SpellPowerWalk
SpellRinsingBath
SpellRudeOutrage
SpellRudeOutrageInflict
SpellSlipperyCold
SpellSlipperyColdInflict
SpellSmokeBomb
SpellSnakeHeads
SpellSolarOven
SpellSparkles
SpellStayCool
SpellStayCoolInflict
SpellTacticalRainbow
SpellWeakenToCute
SpellWeakenToCuteInflict
SpellWeakenToFire
SpellWeakenToFireInflict
SpellWeakenToIce
SpellWeakenToIceInflict
SpellWeakenToNasty
SpellWeakenToNastyInflict
Resource lists – Music
All the music in the base game. Used with the music: command among others.
BattleCheeseA
BattleCheeseB
Castle
Celebration
Clock
Credits
Eerie
Employment
FunnyCharacter
GameShow
Hospital
OverworldEarly
OverworldMid
OverworldLate
OverworldEnd
PlotThickens
Pompous
SereneHappy
SereneRevelation
Setup
Shop
ThemeUndoneShort
TimeUp
Title
Resource lists – Jobs
All the jobs in the base game.
Resource lists – Job skills
All the job skills in the base game.
Resource lists – Weapon skills
All the weapon skills in the base game.
Resource lists – Accessory skills
All the accessory skills in the base game.
Resource lists – Weapons
All the weapons in the base game.
(Fighting spirit)
Resource lists – Armors
All the armors in the base game.
Resource lists – Accessories
All the accessories in the base game.
Resource lists – Items
All the event and quest items in the base game. These items do nothing and cannot be sold in shops.
Resource lists – Spells
All the non-chaos spells in the base game.
Resource lists – Chaos spells
All the chaos spells in the base game. These cannot be found in shops, and are instead obtained from special events that will not appear for the player in first place.
Whether a chaos spell is major or minor simply determines which reward table the belong to. If it is “None” it does not appear in any reward tables and must be given as a specific reward.
Resource lists – Followers
All the followers in the base game. These are given to players with +item: as if giving them an item. Each one has a followerskill which has the same name as the follower itself, and has the listed effect.
Resource lists – Monsters
All the monsters in the base game.
Level 1 random monsters (Region 1)
bugonslug
carnivorouspal
fastbird
lazyshroom
trashmob
Level 5 random monsters (Region 2)
birdsassin
dancemacabre
doublebird
firesnout
flopflop
rogueroguerogue
roughrougerogue
sawbird
spiraltop
toughfishbun
Level 10 random monsters (Region 3)
apatheticmage
babymotorkobra
burrowingcreature
carnivorous_couch
crybulb
deathmask
feralbicycle
hawklike
mockeryofnature
motorkobra
northernwind
rowbugs
Level 15 random monsters (Region 4)
chiller
firefly
furnaceman
hypesnake
kaisernova
manpion
migranite
passingomen
pieceofcake
pteranomancer
pumpingbug
sleepparalysis
snakeman
specterofdeath
Level 5 special monsters and midbosses
scaryscroll
terriblytemperedtome
totalenforcers
wreckingrider
Level 10 special monsters and midbosses
avengingspirit
mainquest_boss_icelady
mainquest_boss_shapestealer
mainquest_boss_ufo
wail
Level 15 special monsters and midbosses
arenaowner
bagofsorrows
goldenspacenord
harvestmaiden
hypedra
mainquest_boss_annebiguous
mainquest_boss_kallahanden
totalenforcers2
werehouse
Level 20 special monsters and midbosses
ultrafishbunjin
Level 15 final bosses (has the “boss” and “skip_ending” tags)
mainquest_boss_captor
mainquest_boss_moosecles
mainquest_boss_weirdo
Level 20 final bosses (has the “boss” and “skip_ending” tags)
mainquest_boss_crowncourt
mainquest_boss_formless
mainquest_boss_formlessfake
mainquest_boss_frostwrack
mainquest_boss_furnacelord
lingeringlivid_00ma00md – Magic attack, Magic defense
lingeringlivid_00mapd00 – Magic attack, Physical defense
lingeringlivid_00mapdmd – Magic attack, Physical defense, Magic defense
lingeringlivid_pa0000md – Physical attack, Magic defense
lingeringlivid_pa00pd00 – Physical attack, Physical defense
lingeringlivid_pa00pdmd – Physical attack, Physical defense, Magic defense
lingeringlivid_pamapdmd – Physical attack, Magic attack, Physical defense, Magic defense
Resource lists – Reward tables
These are the most common reward tables you’ll want to use in event scripting.
The special case “+reward: #jobarea” gives a player a random piece of equipment (weapon, armor or accessory) that matches the current region and the player’s current job. This is determined by the tag “wanted_by”, as seen in the resource tables above.
The following tables include all gear that isn’t marked as “Only found in events” or “Only found with trashcollector skill” in the resource lists:
The following tables include all gear that isn’t marked as “Only found in events”, “Only found with trashcollector skill”, or “Not found in shops” in the resource lists:
The following tables include only the best gear in each region that isn’t marked as “Only found in events” or “Only found with trashcollector skill” in the resource lists:
The following are tables of spells:
The following are auto-generated tables:
The following are custom tables used by various events in the base game:
t1_trashcollection
t2_trashcollection
t3_trashcollection
t4_trashcollection
blackmarket_weapon
luxurywheel_weapon
t4_snok_weapon
blackmarket_armor
luxurywheel_armor
blackmarket_relic
luxurywheel_relic
t2_shopclean
t3_shopclean
t1_itemchest
t2_itemchest
t3_itemchest
t4_itemchest
t1_pittrap
t2_pittrap
t3_pittrap
t4_pittrap
ludo_set
harvestmaiden_reward
t2_vending_equip
t3_vending_equip
t4_vending_equip
sleepyfairymagic
Design – Event rewards
Here are the typical rewards used in the base events and scenarios.
the main reward is something else
Design – Monsters
These are approximate values for enemies and bosses throughout the game. You don’t need to follow these guidelines, but it’ll help balance the monsters against the player’s power level as they progress through the scenario.
Note for Lvl 10 Midbosses:
- The table above only shows the Exp for the monster itself. The Main Quest event typically adds 900 Exp as a reward on top.
Notes for Lvl 20 Final bosses:
- It’s important that final bosses are strong against the Nasty element, since instant KO spells are Nasty-aligned.
- There is also the Cute-aligned spell Hug to Death, which is not normally obtainable except from fighting Formless, who is strong against Cute so you can’t obtain the spell and use it on her.
- Final bosses typically do not award Exp. Instead the game awards Exp in the ending based on how much damage each player dealt to the boss, according to the scenario’s boss_exp_multiplier value.
- Use the tag “boss” for a final boss to give it a unique background and music.
- Use the tag “skip_ending” for a final boss to skip the normal battle ending that plays a fanfare and gives you rewards, if you want your event to handle that instead.
Thanks to Kataiser, hahu, and dooeyjo for their excellent guide on how to mod, all credits belong to his effort. if this guide helps you, please support and rate it via Steam Community. enjoy the game.